Serial Peripheral Interface (SPI)
-
a Serial Data In (SDI), on which the controller sends data to the peripheral devices.
-
a Serial Data Out (SDO), on which the peripheral devices send data to the controller.
-
a Clock (SCLK) connection, on which the controller sends a regular clock signal to the peripheral devices.
-
one or more Chip Select (CS) connections, which the controller uses to signal the peripheral devices when to listen to incoming data and when to ignore it.

Inter-Integrated Circuit (I2C)
I2C is another popular synchronous serial protocol. It also uses a bus configuration like SPI, but there are only two connections between the controller device and the peripheral devices as shown in Figure 4:
-
a Serial Clock (SCL) connection, on which the controller sends the clock signal, just as in SPI
-
a Serial Data (SDA) connection, on which the controller and peripherals exchange data in both directions.

Lab: OLED Screen Display using I2C

#include <Wire.h>
#include <Adafruit_SSD1306.h>
#include <Adafruit_GFX.h>
const int SCREEN_WIDTH = 128; // OLED display width, in pixels
const int SCREEN_HEIGHT = 64; // OLED display height, in pixels
// initialize the display:
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT);
void setup() {
// initialize serial and wait 3 secs for serial monitor to open:
Serial.begin(9600);
if (!Serial) delay(3000);
// first parameter of begin() sets voltage source.
// SSD1306_SWITCHCAPVCC is for 3.3V
// second parameter is I2C address, which is
// 0x3C, or 3D for some 128x64 modules:
if (!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println("Display setup failed");
while (true);
}
Serial.println("Display is good to go");
}
void loop() {
int sensorReading = analogRead(A0);
// clear the display:
display.clearDisplay();
// set the text size to w:
display.setTextSize(2);
// set the text color to white:
display.setTextColor(SSD1306_WHITE);
// move the cursor to 0,0:
display.setCursor(0, 0);
// print the seconds:
display.print("secs:");
display.print(millis() / 1000);
// move the cursor down 20 pixels:
display.setCursor(0, 20);
// print a sensor reading:
display.print("sensor:");
display.print(sensorReading);
// push everything out to the screen:
display.display();
}
Project 2 tests
soil moisture sensor
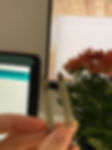
in the air testing
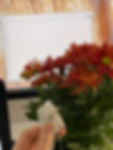
tissue with water testing
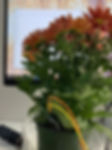
in the soil testing

sensor testing